In this script, we will see how to send emails in Python using the SMTPLIB library. SMTPLIB is a popular python library that is useful to send email via script.
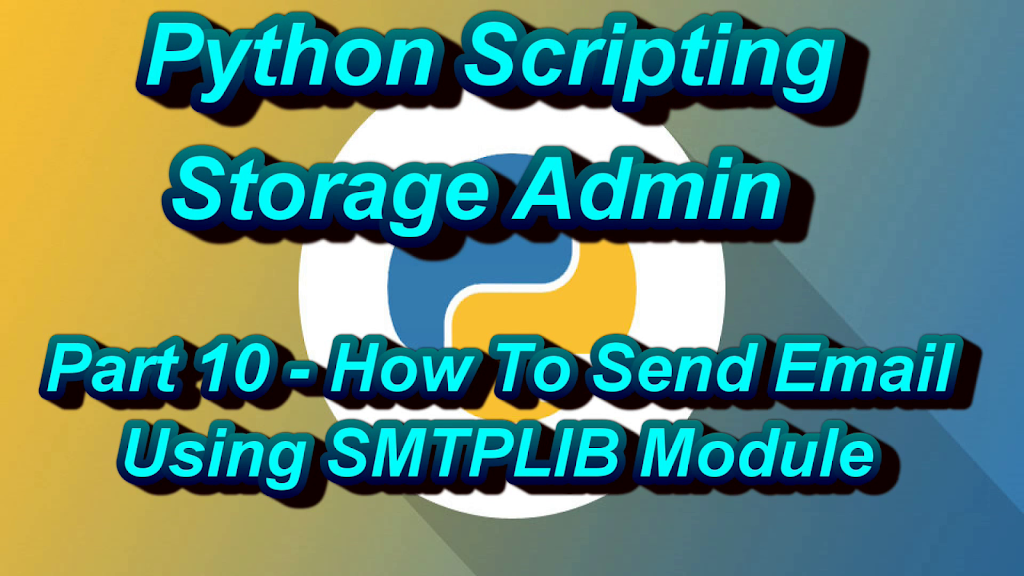
You do not have to install it, because the library comes by default. In the below python script we use Gmail for the demo, but in your case, you have to use your email server.
Before you understand the script, let’s see what are the details you need to send an email in python. Check out the python series for storage admin.
Pre-Requisites To Send Email In Python
In order for the script to work, you need to import the library. As the SMTPLIB library comes by default with python, hence, you do not need to install it.
You also need a SMTP server and ports. You can get them for your team who are responsible for managing the exchange servers.
Once you get the SMTP server and port, make sure that the server where your script will run is allowed to send an email. Usually, a server is allowed to send an email if it is added to the SMTP relay.
Python Scripts to Send Email
Below is the entire script which sends email in python. You just have to modify the SMTP server and port. Notice that the username and password for the Gmail account are saved in the text file. If you are using the script in your company, then you can remove those lines.
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
msg= MIMEMultipart('alternative')
msg['Subject'] = 'Test Mail'
From = "fromemailaddress@gmail.com"
To = "toemailaddress@yahoo.co.in"
Cc = "ccemailaddress@yahoo.co.in"
text = "Info Collector"
data = "Body content of the mail"
part1 = MIMEText(text, 'plain')
part2 = MIMEText(data, 'plain')
msg.attach(part1)
msg.attach(part2)
s=smtplib.SMTP('smtp.gmail.com', 587)
s.ehlo()
s.starttls()
gmail_cred = open("gmail_cred.txt","r").read().split(",")
s.login(gmail_cred[0],gmail_cred[1])
s.sendmail(From, To ,msg.as_string())
s.quit()
If you need more details on the script, then watch the below YouTube video. This video is part of series which explains python scripting for Storage Admin.