In this post, we will see how to read, write and modify files in python script. We can open a file using python script, then we will write some content in the file, and then save it using python script.
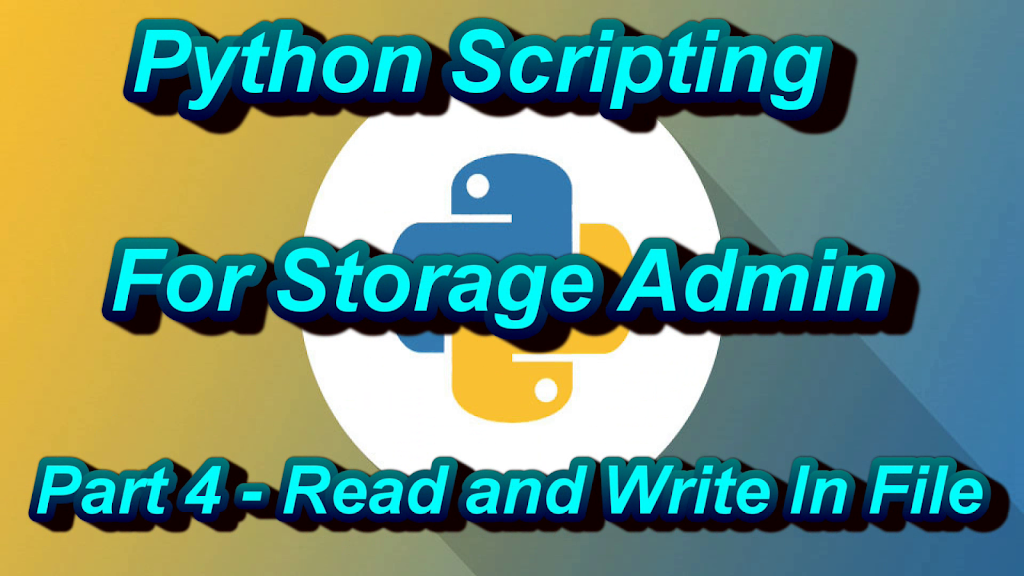
This is also called as file handling in python script. File handling in python deals with all kinds of operations such as open, read, write, and modify files. In the coming section, we will cover all file handling operations using a python script.
Being a Storage Administrator, file handling is very useful while storage automation. One of the most common examples is reading a credential file.
In the coming section, we will see all kinds of operations that we can do with a file using python script.
Open Files In Python
The first thing we can do is to open a file to read. Let’s say you have a credential file, where you have stored the user name and password, and you want to read the file. Below is the sample code you can use to open a file in python script.
s=open(“cred.txt”,”r”)
In the above example, s is an object that we use to open a file cred.txt. Now, it does not do anything, it just opens the file. To read the content you need to use read() function. The below line of code reads the entire content of a file and store the data in variable file_content.
s=open(“cred.txt”,”r”)
file_content=s.read()
print(file_content)
Now, reading the entire content of a file may not be useful. You may need to read the content of a file one by one. The below code will open a file and read the content line by line.
s=open(“cred.txt”,”r”)
file_content=s.readlines()
Now file_content is a list and to print the lines one by one we need to use a for loops.
for lines in file_content:
print(lines)
Write Data In Files Using Python
While doing storage automation, most of the time I use a python script to write the output of commands into a file. The process of writing data in a file is similar to reading files. The only difference is that you need to open the file in write mode.
The below line of code will open a file in write mode and write data to the file. You should always close the file using the close function. If you forget to close, then the data will not be stored in the file.
s=open(“file.txt”,”w”)
s.write(“First line of the file.”)
s.close()
Update Files Content Using Python
Please note that write mode always overwrites the content of a file. Hence, to write additional data in the same, you need to open the file in append mode.
Below line of code will add a new line in an existing file.
s=open(“file.txt”,”a”)
s.write(“Second line of the file.”)
s.close()
Python File Handling Video Tutorial
File handling may be complex for a beginner. Hence, we have made a dedicated video tutorial on it. Watch this video for detail and live tutorials.
Subscribe to the YouTube channel as well and stay tuned for more videos in this video series.